More ways to operate, more possibilities
SDropTarget is used to implement various drag-and-drop operations, such as dragging and dropping files into it to read files or dragging and dropping Actors and other instances into the tool to get instances.
Drag-and-drop operations allow users to interact with tools more intuitively, providing a better experience.
For example, in Object Detail View, we can directly drag and drop the objects we want to view into the window.
In the Shelf tool, we can drag and drop text, Actors, Chameleon Tool tools, etc. into the window.
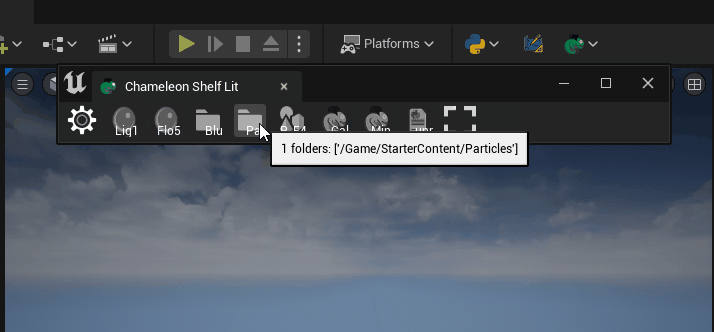
In this video, we can drag and drop images into the editor window, get their path and content, and then perform subsequent operations.
OnDrop¶
For example, in the code below, we put an SMultiLineEditableTextBox in an SDropTarget, and when files or text are dragged and dropped into the SDropTarget, the on_drop_func function will be triggered, thus obtaining the files or text dragged and dropped by the user.
"SDropTarget": {
"Text": "SDropTarget",
"HAlign": "Center",
"OnDrop": "chameleon_instance.on_drop_func(%**kwargs)",
"Content":
{
"SMultiLineEditableTextBox":
{
"Aka": "file_path_input",
"Text": "Drag and Drop, File or Text"
}
}
}
Supported Types¶
- Assets. UAssets in Unreal.
- Folder. Folders in Content Browser.
- Actors. Actor instances.
- External file. For instance, drag and drop a file from windows explorer.
- External text. Select some text in Text Editor(Pycharm, sublime, notepad++), and drag and drop it.
Variable Placeholders¶
%**kwargs¶
In the JSON file above, "chameleon_instance.on_drop_func(%**kwargs)"
. We used the %**kwargs
variable placeholder. At runtime, it will be replaced with the actual value.
For example:
When we drag and drop a file into the SDropTarget, %**kwargs
will be replaced with: files = [file_path_a, file_path_b_if_exists, ...]
,
When dragging and dropping text, %**kwargs
will be replaced with: text = text_content
In this way, we can capture all parameters and their corresponding values in on_drop_func
through **kwargs
.
The specific parameters currently used are:
- "assets"
- "assets_folders"
- "folders"
- "text"
- "files"
Corresponding to the five supported object types above.
TIP
You can get the currently available parameter names through kwargs.keys().
def on_drop_func(self, *args, **kwargs):
file_names = kwargs.get("files", None)
text = kwargs.get("text", "")
if file_names:
file_path = file_names[0]
print(f"file_path: {file_path}")
if os.path.exists(file_path):
self.data.set_text(self.ui_file_path_input, file_path)
else:
if text:
print(f"text: {text}")
self.data.set_text(self.ui_input, text)
Others¶
The following three are legacy variable placeholders. Since their functions overlap with %**kwargs
, they are no longer recommended for use.
- %assets_folders
- %folders
- "%actors
Conclusion¶
SDropTarget is a very useful widget that allows users to interact with tools more intuitively, providing a better experience. Through %**kwargs
, we can obtain the objects dragged and dropped by the user, and then perform subsequent operations in Python.