The most common methods for executing Python commands in Unreal Engine include: command line, menu, tool interface, and Remote Control.
Execute in the Output Window¶
We can execute Python commands through the command line directly in the Output window of the Unreal Engine editor. When debugging and exploring the UE Python editor interface, it's common to operate directly on the command line.
Python and Python(REPL)¶
A REPL (say it, “REP-UL”) is an interactive way to talk to your computer in Python. To make this work, the computer does four things:
- Read the user input (your Python commands).
- Evaluate your code (to work out what you mean).
- Print any results (so you can see the computer’s response).
- Loop back to step 1 (to continue the conversation).
Because we can iterate and progress continuously in the loop of these four steps in the REPL, we can learn through exploration, just like a child discovering all sorts of interesting things in the forest for the first time. For more on learning and iteration, see here: learning_ue_with_python
Executing via the menu¶
When using TAPython, we can mount any Python code on a menu item. This can be a single statement, multiple lines, or an entire Python file. The supported mountable menu item locations can be found here
In fact, the code following command: is almost identical in effect to executing it in the Output window.
{
"OnMainMenu": {
"items": [
{
"name": "TAPython Example: Hello World",
"command": "print('Hello world.')"
}
]
}
}
Or executing a function which in a tool package.
"OnMainMenu": {
"items": [
{
"name": "Call Function",
"command": "import Utilities; print(Utilities.Utils.get_selected_asset())"
}
]
}
NOTE
The code within "command" is executed within the global namespace (similar to the Python command line in the Outline window).
This means that if you define a variable within "command", it will be a global variable.
TIP
We can use print(globals()) to print out the names and values of all global variables.
Using the TAPython Unreal API.¶
ExecPythonCommand¶
In addition to the above methods, we can also call Python code through the extended EditorAPI.
unreal.PythonBPLib.exec_python_command("some_global_var = 'some value'")
Similarly, the code executed within the extended EditorAPI is also executed within the global namespace.
For example, the following code takes advantage of this feature and connects two different ChameleonTool tools together:
def subscribe_macropad_input(self):
if not self.keypad:
self.keypad = MacroPad.MyKeyPad(button_count=14, start_note=60, rotary_count=2)
self.keypad.add_key_callback(id=8, on_key_down=self.create_grass_around_cam)
self.keypad.add_key_callback(id=9, on_key_down=self.toggle_smalls)
unreal.PythonBPLib.exec_python_command("chameleon_midi.add_subscriber(chameleon_zelda_map.keypad)") # self == chameleon_zelda_map
Extra
`chameleon_midi is a tool responsible for interacting with the physical keyboard shown in the figure below, while self is a scene control tool. subscribe_macropad_input associates the specific execution logic in self with the physical keyboard through the observer pattern.
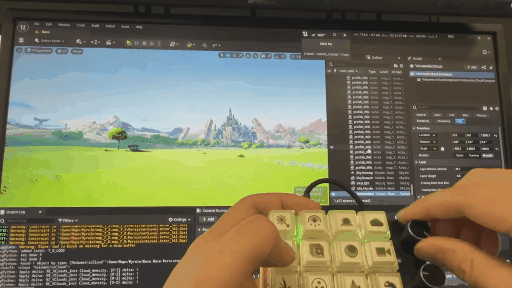
ExecuteConsoleCommand¶
The official documentation introduces running Python script files via the Cmd command line using py "some_py_file_path.py". In TAPython, unreal.PythonBPLib.execute_console_command provides the functionality to execute UE Cmd command lines in Python, so the following code is equivalent to executing it in a Cmd window. (In practice, it's relatively rare to run a .py file using this method.)
unreal.PythonBPLib.execute_console_command('py "some_py_file_path.py"')
Shelf¶
In the Built-in tool, the Shelf tool allows us to drag and drop Python code snippets into the tool (yes, this is a function similar to Maya's shelf, which I really like: D).
In the current version of "Shelf", Python commands are actually executed through unreal.PythonBPLib.exec_python_command.
Remote Control¶
UE Python Remote Control is a powerful feature that allows us to send and execute remote control commands to the UE editor from external software or other Python projects. For example, we can send instructions to import an FBX file and create a model in the scene directly from a DCC software.
This can be seen as a low-end version of live-link, which can be used in DCC or other software without UE live-link.
Other¶
Unreal Engine added the ability to run Python menus through configuration menus in 4.23, using the Customized ToolMenuEntry method.
Compared to this, using TAPython is simpler and more efficient, and is also compatible with the Anchor attachment points that ToolMenuEntry can use.
REPL:(Read Eval Print Loop)