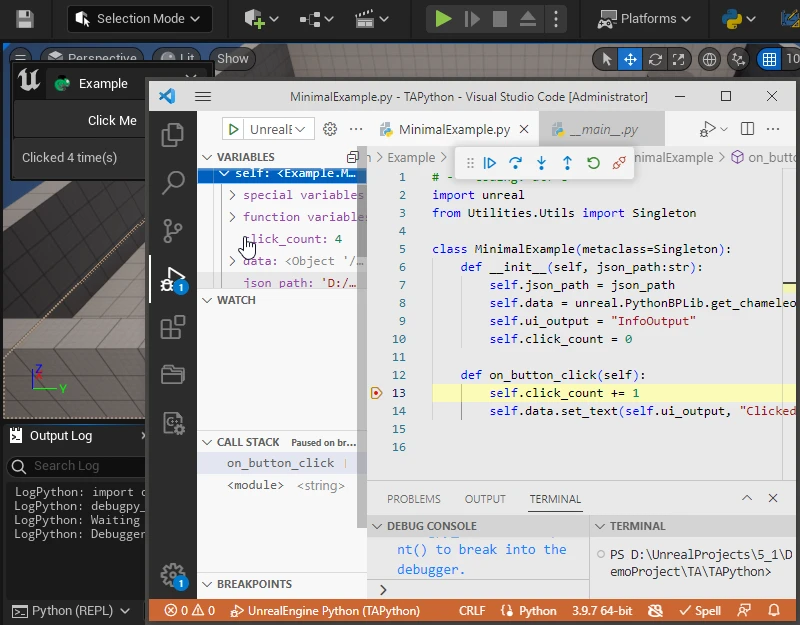
当需要调试python代码时,可以通过以下步骤在VSCode中进行断点调试。 官方python插件在<UE_Root>\Engine\Plugins\Experimental\PythonScriptPlugin\Content\Python\debugpy_unreal.py
中也有步骤说明:
- import debugpy_unreal into Python within the UE Editor.
- If debugpy has not yet been installed, run debugpy_unreal.install_debugpy().
- Run debugpy_unreal.start() to begin a debug session, using the same port that you will use to attach your debugger (5678 is the default for VS Code).
- Attach your debugger from VS Code (see the "launch.json" configuration below).
- Run debugpy_unreal.breakpoint() to instruct the attached debugger to breakpoint on the next statement executed.
步骤¶
- 通过pip 安装第三方库. Use 3rd Python package
- 在VSCode中添加调试配置文件:主菜单-运行-添加配置-Python-远程连接,主机名:localhost, 端口:5678, redirectOutput: true
{
"version": "0.2.0",
"configurations": [
{
"name": "Unreal Python",
"type": "python",
"request": "attach",
"connect": {
"host": "localhost",
"port": 5678
},
"redirectOutput": true
}
]
}
- 当需要调试时,在UE的python的命令行控制台中运行
import debugpy_unreal
debugpy_unreal.start()
debugpy_unreal.start()中会调用 debugpy.wait_for_client()等待 VSCode,此时会表现为编辑器卡死,不用在意,继续步骤
- 在VSCode中主菜单-运行-启用调试(F5),将VSCode Attach到UE的python中。此步骤确保启用了步骤2中创建的调试配置文件
- 在VSCode中打开需要调试的py文件,设置断点,运行即可。也可在在py文件中通过
debugpy_unreal.breakpoint()
直接中断python的运行。(通过此方法中断的py文件,会自动的在VSCode中打开)
其他¶
在实际开发中,除了在VsCode中调试以外,也会通过以下一些方法,快速排除错误
print大法¶
通过print
或者unreal.log
打印变量的值,可以快速的排除错误。
NOTE
python 中的logging模块,可以用作log输出,但是在UE中, 等显示级别会有问题。例如warn
会以红色的Error显示
直接在编辑器中获取对象¶
python中的对象,不管是unreal
中的对象,还是self
中的对象,比如self.data
,都可在Unreal Engine的Python命令行控制台获取和查看其属性。
比如,Get Object in Unreal Engine Editor 中将各种UObject对象赋值给全局变量_r
,然后查看和其中的属性和方法。
又如,如果想直接修改/获取界面中的内容,也可以在Python命令行中进行。下面的例子,就直接通过chameleon_example
这个全局变量名,获得了最小范例中的Chameleon Tools对象,然后修改了其中的TextBlock的内容。
chameleon_example.data.set_text("InfoOutput", "Some Text")
合理的断言¶
适当得在Python代码中添加断言可以帮助开发者在代码中快速发现错误。
例如,确保对象非空
assert my_object, f"my_object is None."
例如,确保变量的长度,类型等符合预期和要求
assert len(object_pair) == 2, f"object_pair len: {len(object_pair)} != 2"
等等